Indexing on database to increase performance and it’s trade-offs.
In database , indexing is a technique used to improve the speed of the data retrieval operations on a table at the cost of additional storage and maintenance. An index is created on one or more columns of a table and allows the database to find rows much faster than it could without index. Here’s a detailed look at how indexing improves performance and the trade-offs involved:
Scenario
Imagine you manage a large library with thousands of books. You want to create a system that allows users to quickly find books based on various criteria such as title, author, and genre.
Without Indexing
Suppose you have a table called Books:
CREATE TABLE Books (
book_id INT PRIMARY KEY,
title VARCHAR(255),
author VARCHAR(255),
genre VARCHAR(50),
publication_year INT
);
The table might have entries like:
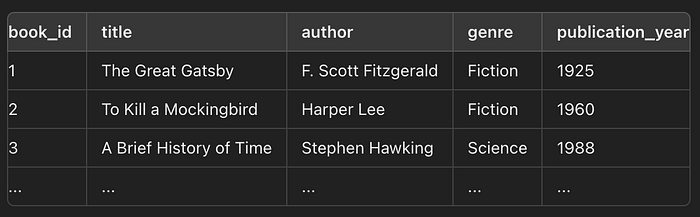
Problem
A user wants to find all books written by “Harper Lee”. Without an index, the database must perform a full table scan to find the matching books, which can be slow for large tables:
SELECT * FROM Books WHERE author = 'Harper Lee';
Solution: Adding an Index
To speed up this query, you can create an index on the author column:
CREATE INDEX idx_author ON Books (author);
How the Index Works
- Index Structure: The index on author will store the values of the author column along with pointers to the corresponding rows in the Books table.
- Efficient Search: When a user searches for books by “Harper Lee”, the database can quickly locate the rows with author = ‘Harper Lee’ using the index instead of scanning the entire table.
Daily Life Analogy
Think of the library’s card catalog system (used before digital databases).
- Without Indexing: Finding a book by author would require you to look at every book on every shelf until you find the one you’re looking for.
- With Indexing: The card catalog (sorted by author) allows you to quickly locate the card for “Harper Lee” and find the exact shelf and position of the book, saving a lot of time.
Further Enhancements
You can create additional indexes for other frequently queried columns, like title and genre:
CREATE INDEX idx_title ON Books (title);
CREATE INDEX idx_genre ON Books (genre);
How Indexing Increases Performance
- Faster Data Retrieval: Indexes allow the database to locate data without scanning the entire table. For example, finding rows where a specific column matches a given value can be done quickly with an index.
- Efficient Sorting: Indexes can speed up sorting operations. If a query involves an ORDER BY clause, the database can use an index to fetch the data in the desired order directly.
- Improved Join Performance: Indexes on columns used in JOIN operations can significantly reduce the time it takes to merge tables by quickly locating matching rows.
- Reduced I/O Operations: By minimizing the need to scan entire tables, indexes reduce the number of disk I/O operations, leading to faster query execution.
Composite Index Example
If users often search for books by both author and genre, you can create a composite index:
CREATE INDEX idx_author_genre ON Books (author, genre);
This composite index would speed up queries like:
SELECT * FROM Books WHERE author = 'Harper Lee' AND genre = 'Fiction';
Unique Indexes
A unique index ensures that all values in the indexed column(s) are distinct. This is useful when you want to enforce uniqueness constraints on certain columns in a table. Let’s consider the library system example again and see how unique indexes can be applied.
Scenario
We want to ensure that each book’s ISBN (International Standard Book Number) is unique in the Books
table. An ISBN is a unique identifier for books, so no two books should have the same ISBN.
Table Definition
Here’s the Books
table, now including an isbn
column:
CREATE TABLE Books (
book_id INT PRIMARY KEY,
title VARCHAR(255),
author VARCHAR(255),
genre VARCHAR(50),
publication_year INT,
isbn VARCHAR(20) -- Assuming ISBNs are stored as strings
);
Adding a Unique Index
To ensure that each isbn
value is unique, you can create a unique index on the isbn
column:
CREATE UNIQUE INDEX idx_unique_isbn ON Books (isbn);
Benefits of a Unique Index
- Enforcing Uniqueness: The database will enforce the rule that no two rows can have the same
isbn
value. This helps maintain data integrity. - Performance: Unique indexes can also improve the performance of queries that search by
isbn
, as the index allows for fast lookups.
Indexes on Expressions
Indexes on expressions, also known as function-based indexes, allow you to create indexes on expressions or computed columns rather than just the raw column values. This can be particularly useful when queries frequently involve operations on columns, such as applying functions, arithmetic, or concatenation.
Why Use Indexes on Expressions?
- Optimizing Complex Queries: When queries frequently use expressions, creating an index on those expressions can significantly speed up query performance.
- Support for Derived Data: Allows indexing on derived data without needing to store additional columns in the table.
- Efficient Query Execution: Helps the database optimizer choose the best execution plan by leveraging the indexed expressions.
Example Scenario
Let’s extend our library system example and consider that users often search for books by a case-insensitive search on the title.
Without an Expression Index
A typical case-insensitive search on the title
column might look like this:
SELECT * FROM Books WHERE LOWER(title) = 'the great gatsby';
Without an index on LOWER(title)
, this query requires a full table scan, which can be slow for large tables.
Creating an Index on an Expression
You can create an index on the expression LOWER(title)
to optimize this query:
CREATE INDEX idx_lower_title ON Books (LOWER(title));
Usage in Queries
Now, the query optimizer can use the index on LOWER(title)
to speed up the search:
SELECT * FROM Books WHERE LOWER(title) = 'the great gatsby';
Trade-offs of Indexes on Expressions
- Storage Overhead: Indexes on expressions require additional storage, similar to regular indexes.
- Maintenance Overhead: Every time the base column(s) change, the index must be updated, which can impact write performance.
- Complexity: Creating and managing function-based indexes can add complexity to database schema design and query optimization.
Conclusion
Indexes on expressions are a powerful tool for optimizing query performance, especially for complex queries involving computed columns or functions. By creating indexes on commonly used expressions, you can significantly speed up query execution and improve overall database performance. However, it’s important to consider the trade-offs, including additional storage requirements and potential impacts on write performance.
Partial Indexes
Partial indexes are a type of index that only includes a subset of rows in a table, typically defined by a condition or predicate. This can be highly beneficial in scenarios where only a portion of the data is frequently queried, allowing for reduced index size and improved performance.
Why Use Partial Indexes?
- Reduced Storage: Partial indexes only index rows that meet a specified condition, reducing the overall storage requirement.
- Improved Performance: Because the index is smaller and more focused, it can improve query performance for the specified subset of data.
- Optimized Maintenance: Fewer rows in the index mean less overhead for maintaining the index during insertions, updates, and deletions.
Example Scenario
Let’s extend our library system example. Suppose you have a Books
table, and you frequently run queries to find books that are classified as "Science Fiction" and published after the year 2000.
Without a Partial Index
A typical query might look like this:
SELECT * FROM Books WHERE genre = 'Science Fiction' AND publication_year > 2000;
Without an index, this query requires a full table scan, which can be slow for large tables.
Creating a Partial Index
You can create a partial index to optimize this specific query:
CREATE INDEX idx_sci_fi_recent ON Books (title)
WHERE genre = 'Science Fiction' AND publication_year > 2000;
Benefits
- Query Performance: The database can use the partial index to quickly find rows where
genre = 'Science Fiction'
andpublication_year > 2000
, avoiding a full table scan. - Efficient Storage: Only rows that match the condition are indexed, reducing storage requirements.
- Faster Updates: Since fewer rows are indexed, insertions, updates, and deletions that do not match the condition have less impact on the index.
Usage in Queries
Now, the query optimizer can use the partial index to speed up the search:
SELECT * FROM Books WHERE genre = 'Science Fiction' AND publication_year > 2000;
Trade-offs of Partial Indexes
- Complexity: Designing partial indexes requires a good understanding of query patterns and data distribution.
- Limited Scope: Partial indexes are only beneficial for queries that match the specified condition. Other queries will not benefit from the index.
- Maintenance Overhead: While partial indexes can reduce overhead for non-matching rows, maintaining the index still involves some overhead for matching rows.
Conclusion
Partial indexes are a powerful tool for optimizing query performance by focusing on a subset of rows that meet specific conditions. They offer reduced storage requirements and improved performance for targeted queries, but they also introduce complexity and require careful design to match query patterns effectively. By leveraging partial indexes, you can enhance the efficiency of your database operations for specific use cases.
Types of Indexes and Their Uses
- B-tree Index: Most common, used for range queries and sorting.
- Hash Index: Efficient for exact match queries.
- Bitmap Index: Suitable for columns with a limited number of distinct values.
- Full-text Index: Used for text search operations.
- Composite Index: Indexes on multiple columns, useful for complex queries involving multiple conditions.
Trade-offs of Indexing
- Increased Storage Requirements: Indexes require additional disk space. Each index adds to the overall storage footprint of the database.
- Slower Write Operations: Indexes need to be updated whenever data in the indexed columns changes. This overhead can slow down INSERT, UPDATE, and DELETE operations.
- Example: When a new row is inserted into the Customers table, the database must also insert a corresponding entry in the idx_last_name index, adding extra time to the insert operation.
- Maintenance Overhead: Regular maintenance tasks such as rebuilding indexes can be necessary to ensure optimal performance, particularly for indexes that become fragmented over time.
- Complexity in Index Management: Deciding which columns to index requires careful analysis of query patterns. Over-indexing can lead to unnecessary overhead, while under-indexing can result in suboptimal query performance.
- Locking Issues: In some databases, indexes can lead to increased locking, where write operations lock index entries, potentially causing contention issues in high-concurrency environments.
Best Practices for Using Indexes
- Analyze Query Patterns: Identify the most frequent and performance-critical queries to determine which columns to index.
- Use Composite Indexes Judiciously: Create composite indexes for queries that filter based on multiple columns.
- Monitor and Maintain Indexes: Regularly monitor index performance and rebuild or reorganize indexes as needed to maintain efficiency.
- Avoid Over-indexing: Only create indexes that provide a clear performance benefit to avoid unnecessary storage and maintenance overhead.
- Consider Indexing Trade-offs: Balance the benefits of faster read operations against the potential slowdown of write operations and additional storage requirements.
Conclusion
Indexing is a crucial tool for optimizing database performance, particularly for read-heavy applications. By carefully selecting which columns to index and regularly maintaining those indexes, you can achieve significant improvements in query performance. However, it’s important to balance these benefits against the associated trade-offs, such as increased storage requirements and potential impacts on write performance.